TL;DR?
Go to the JQuery releases page and click the link for the latest release (as of right now, 11/26/2022, it is 3.X) called "slim minified".
Copy the code from the popup modal and paste it somewhere in between the <head>
tags. Voila! [note: add the accents in the right spot(s)?]
Table of Contents
What is JQuery?
In simplest terms: JQuery is a Javascript "library". It is a bundled file of Javascript code that web developers can import into their website (either via downloading the JQuery library (file) and hosting it in their own website, or by linking to a version of the file hosted somewhere else and delivered by a CDN) and access in order to make writing Javascript easier, as JQuery has many functions available that allow us to perform Javascript functions with "less code" (though technically JQuery is additional code, since it involves importing and/or downloading a Javascript file), but in terms of how much we as web developers have to write, it cuts it down significantly), and much more readable code.
When you use JQuery, you're using the functions and syntax provided by JQuery, which then performs those actions using Javascript behind the scenes. This allows you to avoid using confusingly-named or otherwise more verbose Javascript code. For example, the following code shows the difference between how you might add a class to an HTML element:
Javascript
Let's say we want to add a class of "pink-mode
" to the following button:
<button id="example-btn">Hi, I'm a button!<br>Nothing will happen if you click me right now!</button>
In Javascript, we would do the following:
var buttonElement = document.getElementById('example-btn');
element.classList.add('pink-mode');
JQuery
In JQuery, we can do it like this:
var buttonElement = $('#example-btn');
buttonElement.addClass('pink-mode');
Notice how much less code is used in the JQuery version. Not only that, but the JQuery version is also more generalized. Notice how when I select the button element using its id $('#example-btn')
, I use the "#
" symbol the same way I would if I was writing CSS. This means that if I wanted to select an element by its class, then I could simply change it to $('.example-btn-class-that-does-not-exist')
* using a period (".
") to denote that I'm attempting to use a CSS class to select an element or elements. If I wanted to simply grab an entire element (or elements), I could simply "pass" the name of that element into the selector like so: $('body')
, and now I have access to the <body>
element
Here it is in action!
<button id="example-btn">Hi, I'm a button!<br>Click me!</button>
<!-- Here I'm going to add the Javascript to my HTML inside <script> tags, but you can always make a Javascript file and import it into your HTML page, too, which is usually preferable as it's more organized and keeps the size of the HTML page down. -->
<script>
// Setting a "variable" (var) to keep track of whether or not the "pink" theme has been applied to my button
var pinkApplied = false;
// I'm also saving the button to a variable so it's more readable as "exampleButton" rather than creating a new reference to the button every time by using "$('#example-btn')"
var exampleButton = $('#example-btn');
// This is JQuery's way of adding an "event listener", which listens for a "click event" (i.e. a user clicking on the targeted button)
exampleButton.click(() => {
// When someone clicks on this button, set the variable that tracks whether or not the theme is applied to the opposite of itself (i.e. true becomes false, and false becomes true)
pinkApplied = !pinkApplied;
if(pinkApplied) {
exampleButton.addClass('pink-theme')
}
});
</script>
Footnotes
*Note that if you do this with a class that is present on multiple elements, JQuery will "return" an array of all HTML elements with that class. Don't worry about this too much right now, but just noting it. :)
Do I Actually Need JQuery?
JQuery makes writing Javascript a lot easier! If you are using, or want to use, or are thinking about using Javascript in your website, then JQuery might be a good fit for you!
How do I know if I want to use Javascript in my website?
Javascript opens up a lot of doors for interactivity in your website, so if you like the idea of having users trigger events by interacting with your page, or you want to add more complex features, Javascript can help you! Imagine someone clicks a button that causes text or images to appear or disappear, for instance), or any number of very useful features for your website, such as adding different "themes", adding a light mode and dark mode that users can select between, adding accessible spoiler text (check out my shrine to the movie In the Mouth of Madness to see the spoiler text that I created using Javascript/JQuery, and the painting at the bottom that changes when you click on it [CW: horror imagery]), etc.
Let's say you wanted to add a "reader mode" or "mobile view" to your website that allows users on mobile devices to more easily navigate your site (check out my old site layout for an example: try clicking the "turn on mobile view" button in the top left). I've noticed a lot of sites on Neocities have notices about not being mobile-friendly, and while I may also make a general guide to building mobile responsive pages, this is another potential solution: giving your visitors an option to simplify or even remove your styling in order to more easily read your content, without having to sacrifice the work you put into your website's layout.
Ok, so do I need JQuery?
If you want to try using Javascript (or you already use it), I would recommend JQuery. JQuery simplifies the process of writing Javascript code and makes it more readable, and it is very low-risk to install. There are also tons and tons of resources for any questions you may have on JQuery or Javascript, so if you've had a problem with JQuery, someone else has too, and the solution is probably a quick search engine query away!
How to Install JQuery
There are two main ways that you can install JQuery on a static website like a Neocities site. Both are extremely easy!
CDN Link in <head>
tag (EASY WAY) (RECOMMENDED WAY)
I recommend this method because it's simpler, and easier to upgrade if you ever want to switch to a different version of JQuery!
To get the link that you'll put somewhere inside your <head>
tags*, just go to the JQuery website and check out their releases page, and look under the heading of the latest release (at the time of writing this on 11/26/2022 it is version 3.X), and select the version you want from the four choices of "uncompressed", "minified", "slim", and "slim minified".
I recommend "slim minified" because it has a smaller file size ("minified"), and "slim" just means it doesn't have a few features of JQuery, or support for certain old deprecated JQuery functions. You can judge for yourself if the features excluded from "JQuery slim" make it worth it for you to use the "minified" link instead (I would not recommend using "uncompressed" or "slim").
Whatever version you want (and feel free to use whatever search engine you like best to find out other people's opinions on which version of the four is the best), click on the name of it (in my case "slim minified"), and a popup modal will appear titled "Code Integration". Copy the code it provides and paste it into your page's <head>
tags! The CDN link it gives you should look something like this:
<script src="https://code.jquery.com/jquery-3.6.1.slim.min.js" integrity="sha256-w8CvhFs7iHNVUtnSP0YKEg00p9Ih13rlL9zGqvLdePA=" crossorigin="anonymous"></script>
Congrats!!! You've installed JQuery! Just remember that if you want to use JQuery on another page in your website, you need to import it using the same link in the head of that page as well!
Footnotes
*The JQuery file needs to be available for your Javascript files (or inline <script>
tags) to access the functions inside it and to use its unique syntax like the $('')
HTML element selector, so it should load first.
Downloading and Hosting JQuery Yourself (STILL PRETTY EASY BUT SLIGHTLY MORE MEDIUM-EASY. MAYBE A 3/10)
Go to JQuery.com/download, and find the latest version you want (as of right now it's 3.X)
Once you've found that version, right click the link and save as. It should save as a .min.js
file, but if it doesn't for whatever reason, you can just click the link, and copy the contents of the Javascript file into your own js file and name it something along the lines of jquery-3.6.1.min.js
(replacing the 3.6.1 if necessary with whatever version you downloaded).
Now you just need to add it to your website by putting that file somewhere in your webite folders. I usually create a Javascript folder, or an "assets" folder where I keep these files, but feel free to put it wherever makes sense to you within your own website files!
Once that's added, you can reference that file on any page of your site the same way you would any other file inside your website. Just add that reference somewhere in between the <head>
tags on the page(s) you want to use JQuery in:
<script src="/javascript/jquery-3.6.1.min.js" type="text/javascript"></script>
Keywords
- Static (adj.): Refers to a website that is delivered to the end user exactly as it is stored. This means that there is little-to-no personalization available to the end user; every user will have the same experience, and cannot alter the experience other users may have (e.g. editing a Wikipedia page alters the experience of other users, as they will also see the same changes when they visit that page. This is not possible in a static webpage).
- End User (noun): The users of a website! So right now, my end user... Is you!
-
CDN (Content Delivery Network) (noun): A Content Delivery Network is pretty much what it says on the tin! It refers to a network that individuals or organizations can request "content" from ("content" meaning "files of various kinds"). As far as JQuery is concerned, we can request the JQuery library file from a CDN via a link such as
https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js
. In this case the G**gle CDN delivers you a minified Javascript file (note the.js
file extension on the end of the link!). -
Minified (adj.): Minified code just refers to code (files) that have been made smaller by a tool. That tool usually takes automated steps to reduce the file size of different file types (in this case Javascript, but css files and others can be minified) by removing things like whitespace (literally " ", spaces) and using as few characters as possible for things like variable names, and function names in Javascript files, decreasing the time it takes a CDN to deliver that file to the website, or for that site to load its own minified file. This is why you sometimes see
.min.
in some file names. -
HTML Tag/HTML Element
(noun): I use "HTML tag" and "HTML element" sort of interchangeably, but a tag is one part of an element. Elements usually have an opening tag, for example, this is the opening tag of a paragraph element:
<p>
And this is an entire paragraph element comprised of the opening tag, content, and closing tag:<p>I'm the content of a paragraph element lol</p>
Links!
Dark Mode?
This is the code used to create the "dark mode" on this page! All it does is add or remove a class from the body element, and change the text of the button! The rest is just CSS.
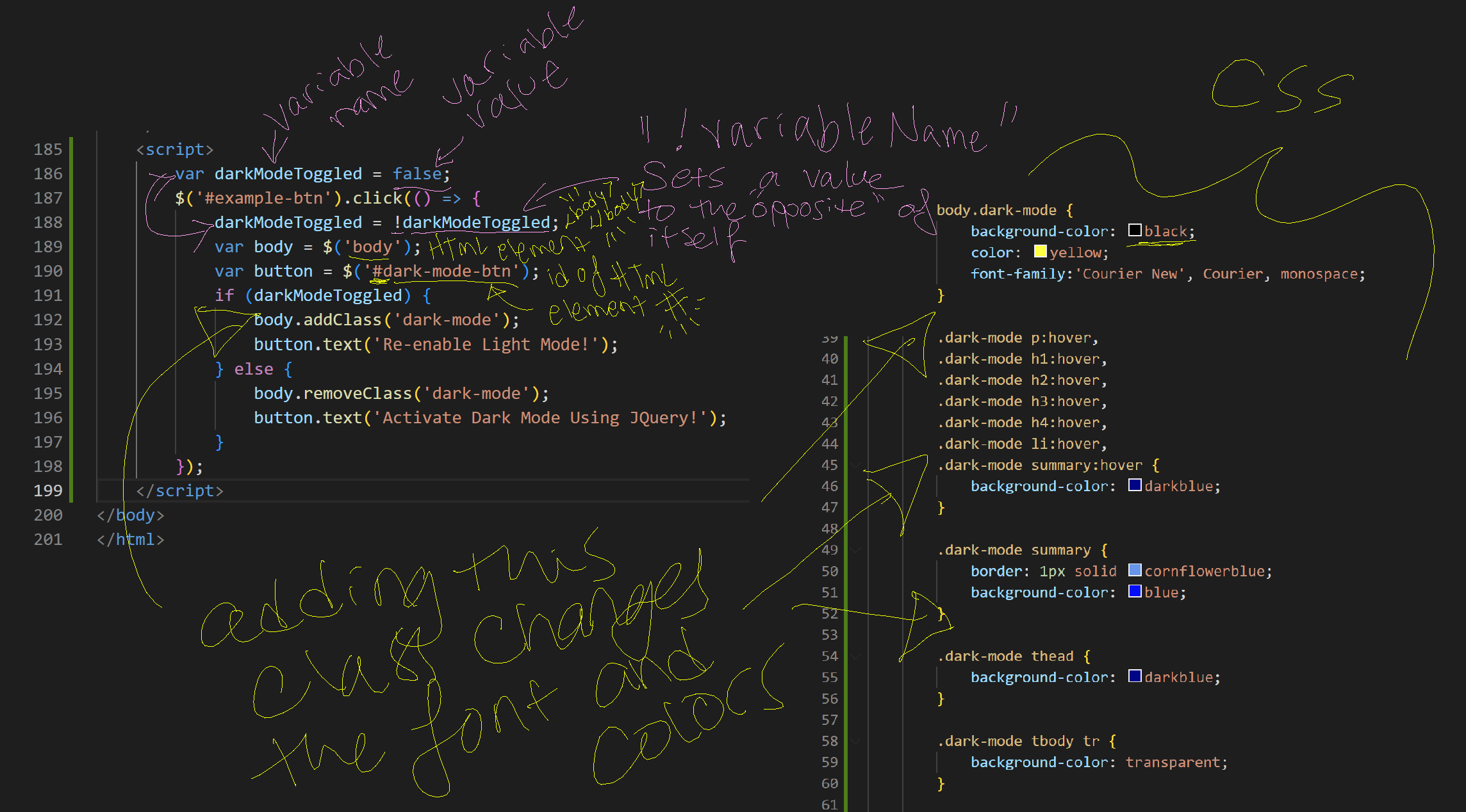
If you want more detailed notes on exactly what each line is doing, feel free to view the source of this page (right click on the page and click "view page source"), and scroll down to the bottom where you should see <script>
tags with the same code inside them with extensive code comments explaining what each line does.